Nader Dabit
- Nov 11, 2021
Building with Arweave, a Permanent, Decentralized Data Protocol
Nader Dabit discusses getting started with building on Arweave, a global, permanent data drive.
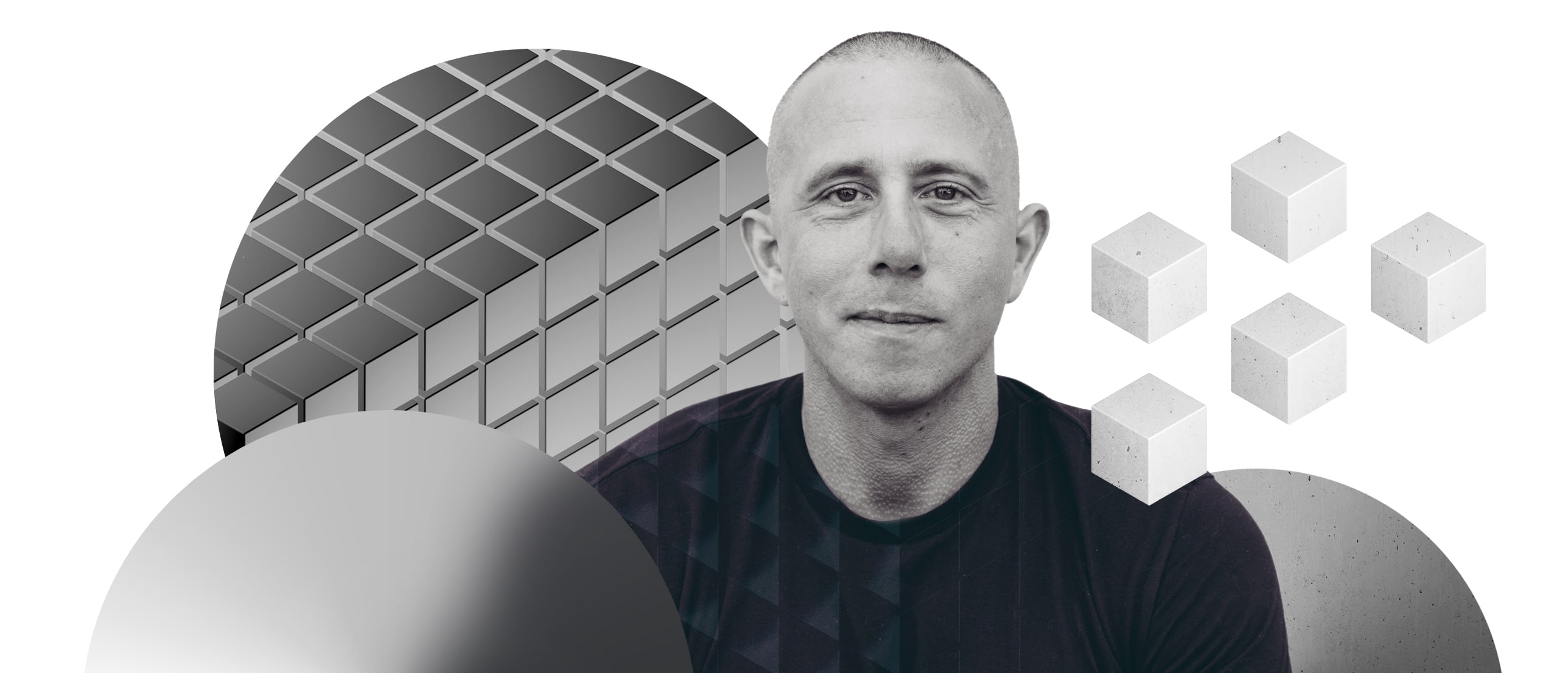
Introducing Building on web3 — a new series of Edge & Node blogs with an aim to familiarize developers with the protocols, tooling, and best practices for developing in the growing web3 space. Building on web3 will spotlight emerging technologies and projects in the web3 ecosystem to help create a recipe for success for dapp developers.
In this post you’ll learn about what Arweave is, why I think it is important, and how you can start building with it. This post will focus on file storage and retrieval, and not on running your own production Arweave gateway.
Arweave is a global, permanent, and decentralized data and file storage layer.
To me, the revolutionary thing about Arweave is the fact that I can store something once and expect that it will be available forever without ever having to pay anything again or without having to worry about it going away.
Compare that to traditional, centralized storage solutions where if you forget to pay your bill one month you get taken down, or even worse, you or someone else accidentally deletes your data completely.
This data permanency is especially important in web3 where we are building immutable application infrastructure. Blockchain data by nature is already immutable, but you often see developers storing off chain data in ways that don’t adhere to decentralization or immutability best practices.
For example in the non-fungible token, or NFT space, some developers use centralized hosting services to store token metadata. This breaks the important security properties required for immutability as well as the idea of what an NFT represents in the first place.
If the metadata is not permanent, there is no guarantee that the property the token represents will still be around in the future. This means that the integrity of the token is at stake if the metadata is not stored on a permanent file storage protocol like Arweave, and why it is so important for technologies like this to exist.
Within The Graph ecosystem, we are also building support and integrations for Arweave because we recognize the value of the protocol for building truly decentralized web3 applications.
About Arweave
Arweave is built on two technologies: the blockweave, a derivative of the blockchain, and ‘Proof of Access’.
Blockweave is the technology that powers Arweave. Like a blockchain, the blockweave is a linked collection of blocks of data, linking to previous blocks written to the network.
The consensus mechanism used to facilitate secure, decentralized data storage in Arweave is called ‘Proof of Access’. This mechanism is more efficient and environmentally friendly than proof of work, because miners do not need to store all blocks. Because of this, an enormous amount of electricity is saved while miners are still able to come to consensus. Also, as the network expands, electricity consumption decreases.
Getting started with Arweave
There are two ways to get started using Arweave tokens
1. Download an Arweave wallet
You can start with a new wallet and get a fraction of Arweave tokens by using the Arweave test faucet.
Once you verify, you should be given 0.1 AR to use to get you started testing out Arweave in your app.
2. A browser wallet
You may have used browser and mobile wallets like Metamask or Phantom that allow you to interact with web3 applications directly from an app. Arweave has a similar wallet, called Arconnect.
You can install this directly as a chrome extension and begin interacting with web applications that use Arweave.
From there, you can fund the wallet with tokens from any of their supported exchanges or you can import your wallet created in the previous step.
To follow this tutorial, you will not need any tokens, but you should have the Arconnect browser wallet installed.
Bundlr
In addition to the technique we’ll cover in this tutorial, you can choose to use Bundlr which which enables native support for Arweave storage on different smart contract blockchains as well as client-side and server-side SDKs for app integration.
This is a great option because it does not require the user to hold AR tokens, but instead can rely on other networks and tokens like ETH, MATIC, Solana, Arbitrum, and many others to pay for transactions.
To learn more about Bundlr, check out the documentation here.
Building with Arweave
Now that we have an overview of Arweave and have secured our tokens, let’s build a basic application.
The app that we will build will allow us to upload and download a string of text to Arweave.
This is a basic example, but a great “Hello world” because it walks through the tools and APIs to get you started building in a short amount of time.
The first thing we’ll do is create a new React application using create-react-app
:
npx create-react-app
arweave-appcd arweave-app
There are two main ways to call the Arweave network from your application:
- HTTP API - The Arweave protocol is based on HTTP so any existing http clients can be used to interact with the network, like Axios or Fetch
- Arweave JS - Arweave JS is the JavaScript/TypeScript SDK for interacting with the Arweave network and uploading data to the permaweb. It works in latest browsers and Node JS.
The Arweave JS SDK is very nice and is what we’ll be using for this tutorial.
Let’s now install arweave-js
using either npm or yarn:
npm install arweave
Arweave network
There are a few ways you can interact with the Arweave network:
- Devnet - arweave.dev
- Mainnet - https://arweave.net/
- Local environment - Arlocal
In this guide, we’ll be starting with Arlocal, which will allow us to send test transactions for free. We’ll also learn how to use mainnet to send a real transaction.
Arlocal
To test locally, we can use Arlocal
, which will run a local network that we can interact with and send test transactions to.
You don’t even need to download or install anything to use Arlocal, as long as you have Node.js installed on your machine, you can just start a local gateway by running:
npx arlocal
That’s it! You should now be running a local Arweave gateway on http://localhost:1984
Now that you have a local gateway running, let’s write the code.
Open src/App.js
and update the file with the following code:
import styles from '../styles/Home.module.css'
import { useState } from 'react'
import Arweave from 'arweave'
/* connect to an Arweave node or specify a gateway */
const arweave = Arweave.init({})
function App() {
const [state, setState] = useState('')
const [transactionId, setTransactionId] = useState('')
async function createTransaction() {
if (!state) return
try {
const formData = state
setState('')
/* creates and sends transaction to Arweave */
let transaction = await arweave.createTransaction({ data: formData })
await arweave.transactions.sign(transaction)
let uploader = await arweave.transactions.getUploader(transaction)
/* upload indicator */
while (!uploader.isComplete) {
await uploader.uploadChunk()
console.log(`${uploader.pctComplete}% complete, ${uploader.uploadedChunks}/${uploader.totalChunks}`)
}
setTransactionId(transaction.id)
} catch (err) {
console.log('error: ', err)
}
}
async function readFromArweave() {
/* read Arweave data using any trsnsaction ID */
arweave.transactions
.getData(transactionId, {
decode: true,
string: true,
})
.then((data) => {
console.log('data: ', data)
})
}
return (
<div className={styles.container}>
<button style={button} onClick={createTransaction}>
Create Transaction
</button>
<button style={button} onClick={readFromArweave}>
Read Transaction
</button>
<input style={input} onChange={(e) => setState(e.target.value)} placeholder="text" value={state} />
</div>
)
}
const button = {
outline: 'none',
border: '1px solid black',
backgroundColor: 'white',
padding: '10px',
width: '200px',
marginBottom: 10,
cursor: 'pointer',
}
const input = {
backgroundColor: '#ddd',
outline: 'none',
border: 'none',
width: '200px',
fontSize: '16px',
padding: '10px',
}
export default App
Next, start the app:
npm start
When the app starts, you should see a basic form input with two buttons.
You can type into the form the data you’d like to save, then press Create Transaction to save the text in Arweave.
Once the transaction is complete, you should see a progress indicator logged out to the console indicating the data has been saved.
Once the data is save, click Read Transaction to read the data from Arweave.
Arweave production network
To interact with the production network, you only need to update the Arweave network configuration.
const arweave = Arweave.init({
host: 'arweave.net',
})
Code for this project
An example codebase for the project we built today is available here.
Next steps
To learn more about Arweave, check out the Arweave docs, the Arweave Wiki, and follow them on Twitter.
About Edge & Node
Edge & Node is a creative software development company working to build a vibrant, decentralized future. Founded by the initial team behind The Graph, Edge & Node is dedicated to the advancement of the decentralized internet (web3) and the proliferation of trust-minimized applications.The team has extensive experience in developing and maintaining open source software, tools, and applications.
Edge & Node’s initial product is The Graph, an indexing protocol for querying networks like Ethereum and IPFS, which ensures open data is always available and easy to access. The Graph is used by thousands of protocols and dapps including Uniswap, Livepeer, Aave, Decentraland, and more. Edge & Node also launched Everest, the first decentralized registry of on-chain data to provide ongoing utility to the crypto community, catalyzing the shift to web3.
To keep up with all things Edge & Node, follow us on Twitter, LinkedIn, and Instagram. Edge & Node is also hiring.
Subscribe to our newsletter for the best of web3
Stay on top of the latest web3 news with a fresh cup of web3 Tea delivered right to your inbox. Web3 Tea is a bi-weekly round-up of web3 developments, macro observations, and profound tweets.
Stay stimulated with web3 Tea, whether you're a web3 beginner or expert!